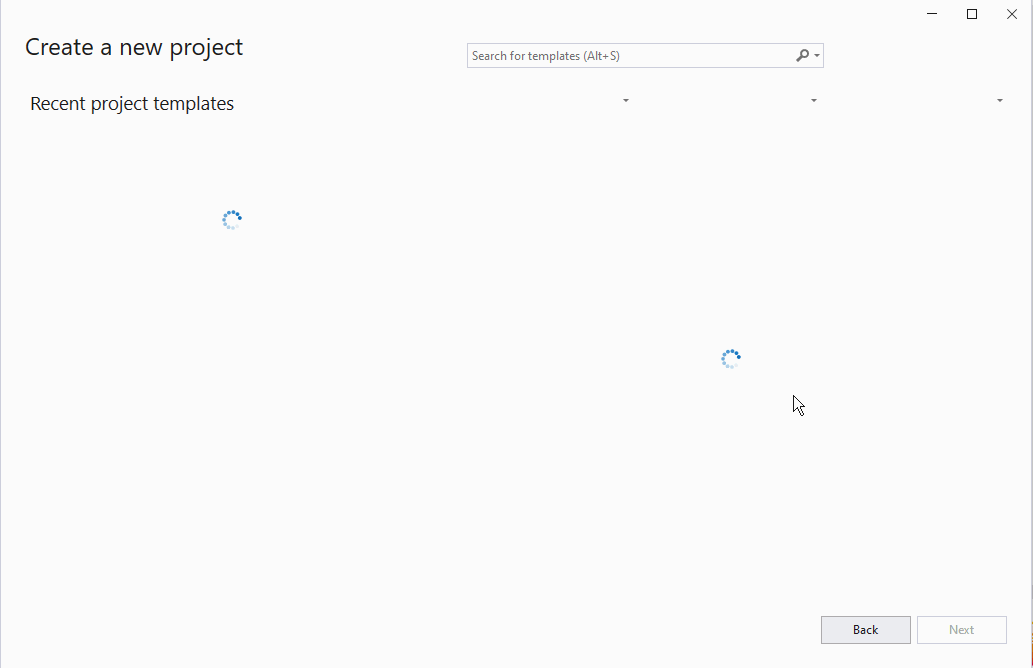
This document attempts to highlight the parts of the C++ language that are most helpful for FRC robotics. Although some of the examples are oriented towards robotics, these lessons do not require any of the FRC software to be installed.
Although there are simpler languages that could be used for robot programming, if you take the time to learn C++ it will be worth the effort. See this blogpost for an excellent argument for learning a lower-level language.
The C++ language is a compiled language which means that a program (a compiler) processes the source code and converts it into an executable file. This allows most errors to be found before the program is actually run. It is possible to use either the Visual Studio IDE (next section) or an online compiler (Online CPP) to work on the practice exercises. Visual Studio allows you to work from your local harddisk which makes it easier to save you work and not lose anything and it works more similarly to the VSCode IDE we use for the robot code. The online compiler (Online CPP) lets you practice coding from any computer connected to the internet which is great if you use a family computer at home and you don’t want to install a (very) large IDE.
An IDE is a system to write, compile, link, debug, and execute code. These tasks (which you probably do not understand yet) are all done from a common application (the IDE). FRC/WPILib uses Microsoft’s Visual Studio IDE. You should install the IDE on a laptop or desktop computer that you will use to learn C++. Follow the directions Visual Studio Community Install and choose the Workload "Desktop Development with C++".
The ubiquitous first program of any programming language is the Hello World program. So ubiquitous in fact that Visual Studio creates the code to output "Hello World" by default when you create a Console Application project.
Launch Visual Studio and select "Create a new project"
Choose a "Console App"
Give it the name HelloWorld
, optionally choose a different location, and check the "Place Solution and Project in the same directory" checkbox
The HelloWorld project is created and the HelloWorld.cpp
is opened in an editor window. All of the lines that begin with double slashes (//
) are comments. The contents of the file with the comments removed is:
#include <iostream>
int main()
{
std::cout << "Hello World!\n";
}
Choose Debug → Start Debugging to run the program. You should see the following output:
Hello World! C:\Users\mylogin\source\repos\HelloWorld\x64\Debug\HelloWorld.exe (process 3356) exited with code 0. To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically close the console when debugging stops. Press any key to close this window . . .
If all of the above goes correctly then you now have a working C++ compiler and can start writing code! If you cannot get this to work, ask for help because this should just work.
There are several good websites for learning C++ online. I will point you in the direction of LearnCPP for a lot of these lessons. The team has a couple of copies of the book "C for Dummies", by Stephen Davis_ that you can use as a secondary resource. If you prefer to learn from a book and want your own copy then I suggest you get _"Programming: Principles and Practice Using C", by Bjarne Stroustrup. It is a really good book but it is a bit expensive.
Each chapter in LearnCPP has several sections and you need to click on the green NEXT LESSON banner near the bottom of each section. There are also quizes for most sections and entire chapter summaries and quizes.
Read Chapter 1: Basic Program Structure
Read Chapter 2: Functions and Files
Write a program that asks the user to enter a number, and then enter a second number. The program should tell the user what the result of adding and subtracting the two numbers is. The output of the program should match the following (assuming inputs of 6 and 4): Enter an integer: 6 Enter another integer: 4 6 + 4 is 10. 6 - 4 is 2. |
|
|
Write a program that has a function that takes two numbers as parameters and returns the ratio of two numbers. It’s function declaration should be:
Take two numbers as user input and print out the ratio of the numbers. Enter a number: 6.0 Enter another number: 4 6.00000 / 4 is 1.50000. |
|
|
Read Chapter 4: Data Types
Read Chapter 5: Constants and Strings
Read Chapter 6: Operators
Read Chapters 8.1 - 8.10: Control Flow
Add an
|
||
|
||
|
Write a program to convert RPMs to radians per second with a function declaration of 500.0000 RPM is 52.3598 rad/s 1000.0000 RPM is 104.71976 rad/s 1500.0000 RPM is 157.07963 rad/s 2000.0000 RPM is 209.43951 rad/s |
The real power of C++ is that it is an object oriented language. The techniques of Object Oriented Programming (OOP) help organize a program by spliting tasks into logical pieces. OOP turns out to be a very powerful way of organizing complex software.
Read Chapter 14: Intro to Classes
Write a The following
Point2d(0.000, 0.000) Point2d(6.000, 5.000) |
Read Chapter 15: More on Classes
Read Chapter 23: Object Relationships
Read Chapter 24: Inheritance
Read Chapter 10: Types and Functions
Read Chapter 26: Template Classes
LearnCPP *